Spreadsheet Dialog
The spreadsheet
dialog allows users to import, preview, and embed spreadsheet data within Uhuu templates.
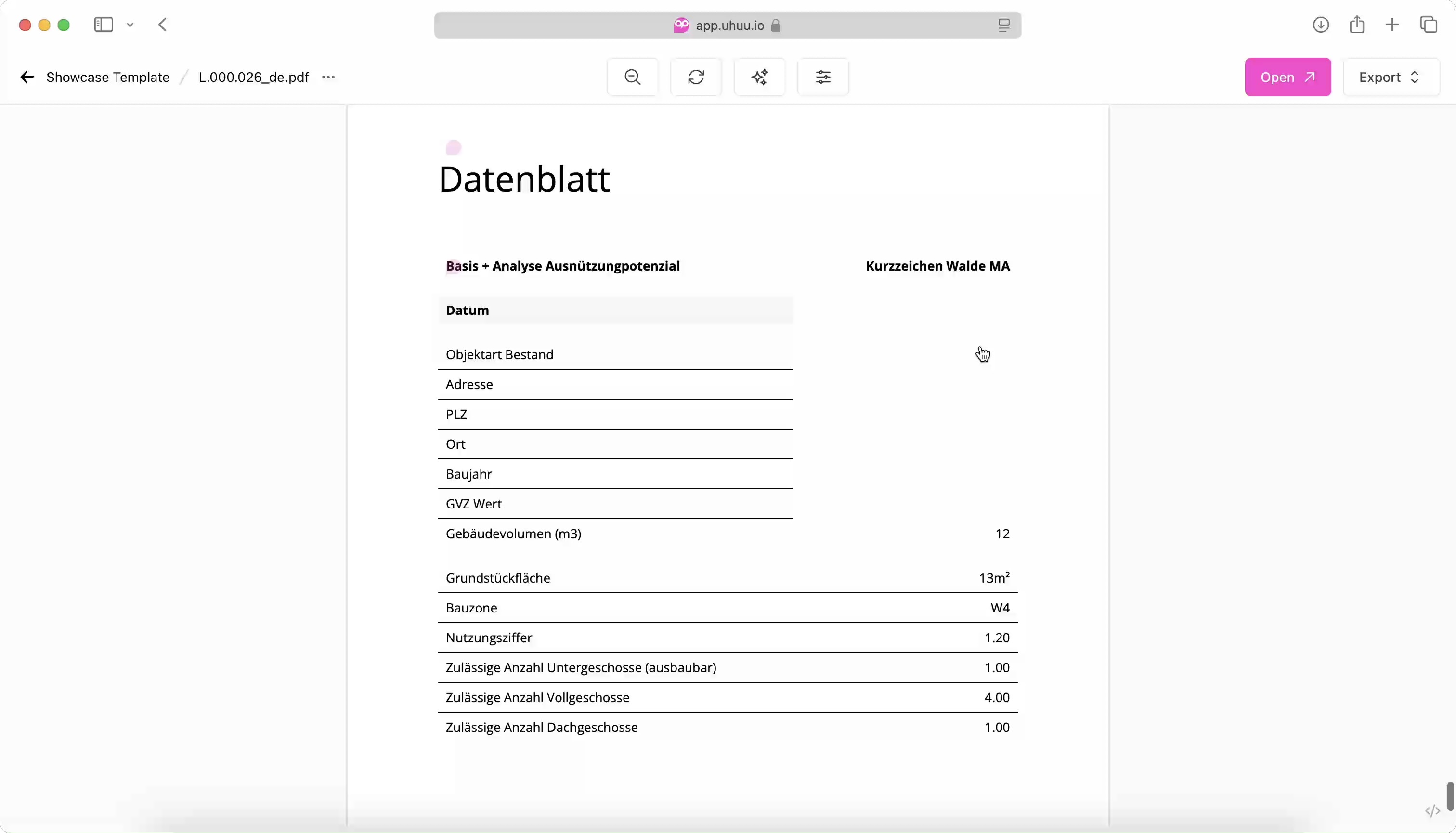
Parameters
The editDialog
method is configured with the following options for spreadsheet editing:
type
(enum, required:"spreadsheet"
): Sets the type to"spreadsheet"
to trigger the Spreadsheet Dialog for loading and displaying table data.path
(string, required): Specifies the path within the payload where the spreadsheet data will be stored. In this example,"sheet_data"
is used as the payload path.
Example Usage
Below is a sample React component setup that uses the Spreadsheet Dialog to load and display data as a table.
export default ({ payload }) => {
const { sheet_data } = payload;
return (
<div onClick={() => $uhuu.editDialog({ path: "sheet_data", type: "spreadsheet" })}>
<div className="table-container" dangerouslySetInnerHTML={{ __html: sheet_data?.html }} />
</div>
);
}
This dialog is particularly useful for displaying data in a tabular format, allowing users to incorporate external data from spreadsheet files directly into their document.
Example Response
After the spreadsheet data is imported, response may look like this in the payload:
{
"html": "<table style=\"border-collapse: collapse;border-spacing: 0;\"><colgroup><col style=\"width: 115px;\"><col style=\"width: 120px;\"></colgroup><tr><td class=\"font-bold\">Name</td><td class=\"font-bold\">Surname</td></tr><tr><td class=\"u-border-b u-border-b-1 italic\"style=\"--u-border-b-color:#000000\">Patrick</td><td class=\"u-border-b u-border-b-1\"style=\"--u-border-b-color:#000000\">Mueller</td></tr><tr><td class=\"u-border-b u-border-b-1 u-border-t u-border-t-1\"style=\"--u-border-b-color:#000000;--u-border-t-color:#000000\">Timon</td><td class=\"u-border-b u-border-b-1 u-border-t u-border-t-1\"style=\"--u-border-b-color:#000000;--u-border-t-color:#000000;--u-text-color:#f50000\">Oberholzer</td></tr><tr><td class=\"u-border-b u-border-b-8 u-border-t u-border-t-1\"style=\"--u-border-b-color:#133DE3;--u-border-t-color:#000000\">John</td><td class=\"u-border-b u-border-b-8 u-border-t u-border-t-1\"style=\"--u-border-b-color:#133DE3;--u-border-t-color:#000000\">Doe</td></tr><tr><td class=\"u-border-t u-border-t-8\"style=\"--u-border-t-color:#133DE3\">Jane</td><td class=\"u-border-t u-border-t-8\"style=\"--u-border-t-color:#133DE3\">Doe</td></tr></table>",
"workbook": {"styles":{"WUQW39":{"bl":1},"q8ZirI":{"ff":"Arial","fs":11,"it":1,"bl":0,"ul":{"s":0},"st":{"s":0},"ol":{"s":0},"tr":{"a":0,"v":0},"td":0,"ht":0,"vt":0,"tb":0,"pd":{"t":0,"b":2,"l":2,"r":2},"bd":{"b":{"s":1,"cl":{"rgb":"#000000"}}}},"2PNx4F":{"bd":{"b":{"s":1,"cl":{"rgb":"#000000"}}}},"GENfFP":{"bd":{"b":{"s":1,"cl":{"rgb":"#000000"}},"t":{"s":1,"cl":{"rgb":"#000000"}}}},"77vemq":{"ff":"Arial","fs":11,"it":0,"bl":0,"ul":{"s":0,"cl":{"rgb":"#f50000"}},"st":{"s":0,"cl":{"rgb":"#f50000"}},"ol":{"s":0,"cl":{"rgb":"#f50000"}},"tr":{"a":0,"v":0},"td":0,"ht":0,"vt":0,"tb":0,"pd":{"t":0,"b":2,"l":2,"r":2},"cl":{"rgb":"#f50000"},"bd":{"b":{"s":1,"cl":{"rgb":"#000000"}},"t":{"s":1,"cl":{"rgb":"#000000"}}}},"BRL65n":{"bd":{"b":{"s":8,"cl":{"rgb":"#133DE3"}},"t":{"s":1,"cl":{"rgb":"#000000"}}}},"kvGJZL":{"bd":{"t":{"s":8,"cl":{"rgb":"#133DE3"}}}}},"sheetOrder":["sheet1"],"sheets":{"sheet1":{"id":"sheet1","cellData":{"0":{"0":{"v":"Name","t":1,"s":"WUQW39"},"1":{"v":"Surname","t":1,"s":"WUQW39"}},"1":{"0":{"v":"Patrick","t":1,"s":"q8ZirI"},"1":{"v":"Mueller","t":1,"s":"2PNx4F"}},"2":{"0":{"v":"Timon","t":1,"s":"GENfFP"},"1":{"v":"Oberholzer","t":1,"s":"77vemq"}},"3":{"0":{"v":"John","t":1,"s":"BRL65n"},"1":{"v":"Doe","t":1,"s":"BRL65n"}},"4":{"0":{"v":"Jane","t":1,"s":"kvGJZL"},"1":{"v":"Doe","t":1,"s":"kvGJZL"}}},"columnData":{"0":{"w":114.6171875,"hd":0},"1":{"w":119.64453125,"hd":0}},"columnCount":2,"rowCount":30,"defaultColumnWidth":88,"defaultRowHeight":24,"mergeData":[]}}}
}
Once imported, the spreadsheet data can be displayed as an HTML table within the template.
<div class="table-container">
<table style="border-collapse: collapse;border-spacing: 0;">
<colgroup>
<col style="width: 115px;">
<col style="width: 120px;">
</colgroup>
<tr>
<td class="font-bold">Name</td>
<td class="font-bold">Surname</td>
</tr>
<tr>
<td class="u-border-b u-border-b-1 italic" style="--u-border-b-color:#000000">Patrick</td>
<td class="u-border-b u-border-b-1" style="--u-border-b-color:#000000">Mueller</td>
</tr>
<tr>
<td class="u-border-b u-border-b-1 u-border-t u-border-t-1"
style="--u-border-b-color:#000000;--u-border-t-color:#000000">Timon
</td>
<td class="u-border-b u-border-b-1 u-border-t u-border-t-1"
style="--u-border-b-color:#000000;--u-border-t-color:#000000;--u-text-color:#f50000">
Oberholzer
</td>
</tr>
<tr>
<td class="u-border-b u-border-b-8 u-border-t u-border-t-1"
style="--u-border-b-color:#133DE3;--u-border-t-color:#000000">John</td>
<td class="u-border-b u-border-b-8 u-border-t u-border-t-1"
style="--u-border-b-color:#133DE3;--u-border-t-color:#000000">Doe</td>
</tr>
<tr>
<td class="u-border-t u-border-t-8" style="--u-border-t-color:#133DE3">Jane</td>
<td class="u-border-t u-border-t-8" style="--u-border-t-color:#133DE3">Doe</td>
</tr>
</table>
</div>
You will notice that we use CSS variables for flexible styling, allowing developers to use these colors dynamically without being restricted to just border, text or background color styling.
Here is an example of CSS for the table:
.table-container {
--u-border-b-color: black;
--u-border-l-color: black;
--u-border-r-color: black;
--u-border-t-color: black;
--u-text-color: black;
--u-bg-color: white;
}
/* Uhuu table border CSS */
.table-container th,
.table-container td {
vertical-align: middle;
background-color: var(--u-bg-color);
color: var(--u-text-color);
}
/* Uhuu table border CSS */
.u-border-b { border-bottom: 1px solid var(--u-border-b-color) ; }
.u-border-l { border-left: 1px solid var(--u-border-l-color); }
.u-border-r { border-right: 1px solid var(--u-border-r-color); }
.u-border-t { border-top: 1px solid var(--u-border-t-color); }
.u-border-b-1 { border-bottom-width: 1px;}
.u-border-b-2 { border-bottom-width: 2px; }
.u-border-b-4 { border-bottom-width: 4px; }
.u-border-b-6 { border-bottom-width: 6px; }
.u-border-b-8 { border-bottom-width: 8px;}
FAQ
Q: How can we create subpixel borders for tables to avoid thick lines and gaps in PDFs?
Regular 1px
borders may appear too thick for some document designs, and using 0.5px
often gets rounded up in PDFs. This might prevent us from creating a desired document result after rendering.
Solution: To create true subpixel borders, we use:
- ::before & ::after with
transform: scaleY(0.5);
for top & bottom borders. - box-shadow for left & right borders to avoid rendering issues.
This ensures sharp, clean, and consistent subpixel borders across PDFs.
Example of how to customize the table border for subpixel border
.table-container th,
.table-container td {
background-color: var(--u-bg-color);
color: var(--u-text-color);
}
/* Uhuu table border CSS */
.u-border-b { border-bottom: 1px solid var(--u-border-b-color); position: relative; }
.u-border-l { border-left: 1px solid var(--u-border-l-color); position: relative; }
.u-border-r { border-right: 1px solid var(--u-border-r-color); position: relative; }
.u-border-t { border-top: 1px solid var(--u-border-t-color); position: relative; }
/* Subpixel border handling */
/* Clear default border */
.u-border-b-1 { border-bottom: 0px solid transparent !important; }
.u-border-l-1 { border-left: 0px solid transparent !important; }
.u-border-t-1 { border-top: 0px solid transparent !important; }
.u-border-r-1 { border-right: 0px solid transparent !important; }
/* Add subpixel border */
.u-border-b-1::after { content: ''; position: absolute; bottom: 0; left: -0.5px; right: -0.5px; height: 1px; background-color: var(--u-border-b-color); transform: scaleY(0.5); transform-origin: bottom; overflow: hidden; }
.u-border-t-1::before { content: ''; position: absolute; top: 0; left: -0.5px; right: -0.5px; height: 1px; background-color: var(--u-border-t-color); transform: scaleY(0.5); transform-origin: top; overflow: hidden; }
.u-border-l-1 { box-shadow: inset 0.5px 0px 0px var(--u-border-l-color); }
.u-border-r-1 { box-shadow: inset -0.5px 0px 0px var(--u-border-r-color); }
.u-border-l-1.u-border-r-1 { box-shadow: inset 0.5px 0px 0px var(--u-border-l-color), inset -0.5px 0px 0px var(--u-border-r-color); }