Edit Dialogs Overview
Uhuu Editor provides a range of dialogs for interactive editing of various data types within templates. Each dialog allows users to update specific paths in the document's payload, automatically updating the data and emitting changes back to the template in real-time.
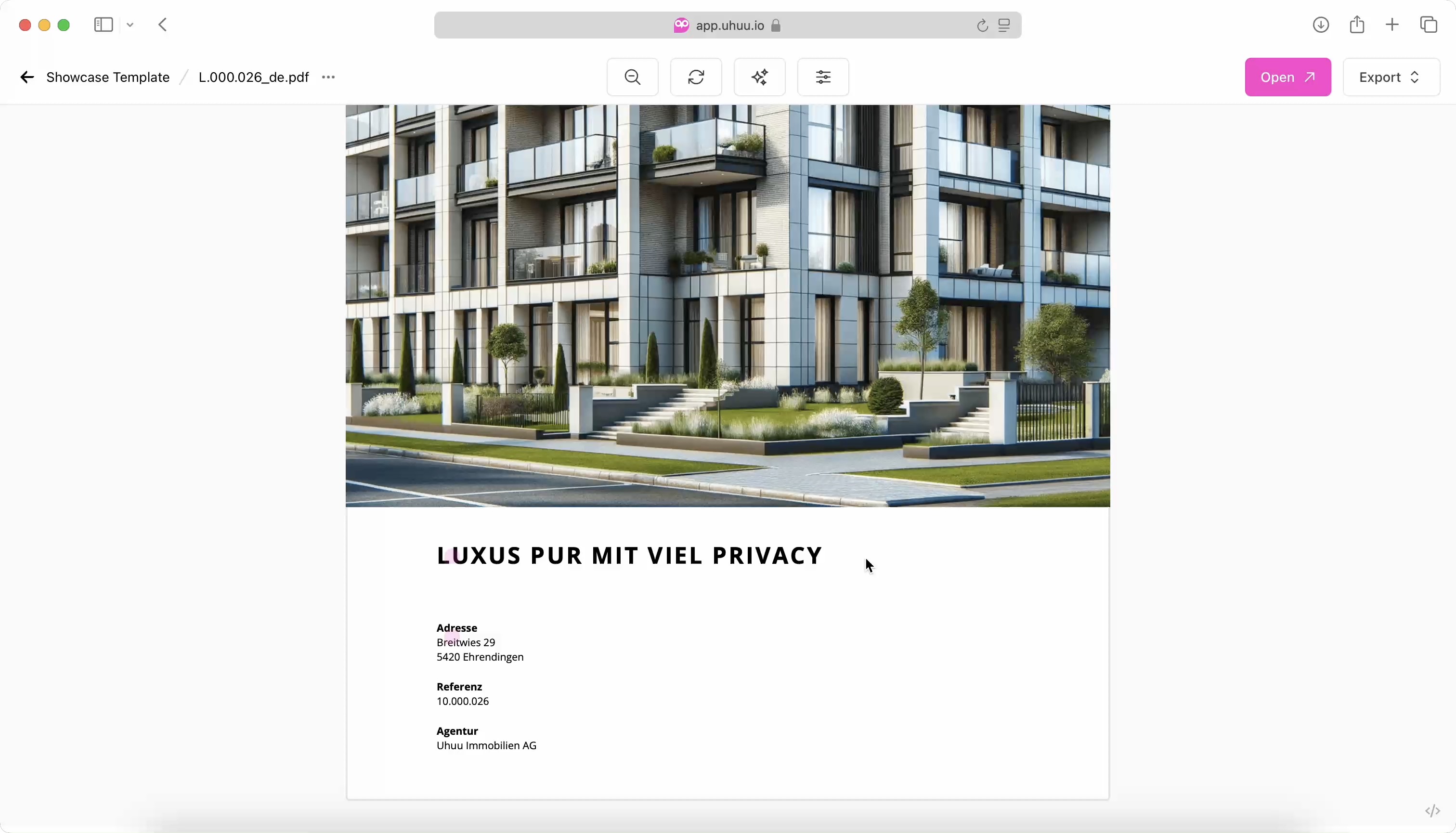
editDialog(options)
The editDialog
method initializes a dialog interaction within the Uhuu editor, enabling users to modify data at targeted paths in the template payload.
Functionality
- Opens a dialog for editing data at a specified path in the payload.
- After saving, Uhuu editor updates the payload with modified data.
- The Uhuu editor updates the payload and emits a
payload
event, allowing templates to listen for changes and re-render or process the updated data.
Parameters
The editDialog
method takes a configuration object with the following base parameters:
type
(string, default:"textarea"
): Specifies the type of dialog. Available types include:textarea
,select
,image
,markdown
,assistant
,dataloader
,spreadsheet
,map
,schemaform
.path
(string, required): The path within the payload where edited data will be stored.value
(optional): Initial content for the specified path when the dialog opens. By default, it retrieves the current value from the payload at the given path.
Basic Dialogs
These dialogs use standard HTML form elements.
Consider the following JSON data for editing:
{
"name": "John Doe",
"icecream": "strawberry"
}
textarea
A multi-line text input.
Parameters
rows
: Specifies the number of rows (default: 3).panelSize
(default:"md"
): Controls the dialog size ("md"
,"3xl"
, or"5xl"
).
Example
$uhuu.editDialog({
path: 'name',
// type: 'textarea' // Can be omitted as 'textarea' is the default type
});
select
A dropdown menu with selectable options.
Parameters
options
: An array of objects for dropdown options, e.g.,[{ value: "chocolate", label: "Chocolate" }]
.
Example
$uhuu.editDialog({
path: 'icecream',
type: 'select',
options: [
{ value: 'chocolate', label: 'Chocolate' },
{ value: 'strawberry', label: 'Strawberry' },
{ value: 'vanilla', label: 'Vanilla' }
]
});
Specialized Dialogs
For more complex data structures, Uhuu provides specialized dialogs:
- Image: Select and edit images within the Uhuu Editor.
- Markdown: Edit text content with markdown formatting.
- AI Assistant: Generate or refine text using AI-based responses.
- Dataloader: Import and load structured data (e.g., CSV) into the template.
- Spreadsheet: Embed spreadsheet data as HTML tables.
- Map: Display and customize location-based data.
- Schema Form: Edit structured data using a JSON Schema-based form.
Each specialized dialog type supports unique configurations for tailored interactions, detailed in the individual dialog documentation.
Multiple Types
When you have multiple dialog types available in an editable area, you can use the types
parameter to pass an array of dialog type options. This lets users choose the preferred way for editing the payload.
Here in the example below, we have two dialog types available for the same field. The first one is a select
dialog type with options. The second one is a textarea
dialog type. When no title is passed, the type is used as default title.
$uhuu.editDialog({
types: [
{ path: 'gelato', type: 'select', options: [{ value: 'chocolate', label: 'Chocolate' },{ value: 'vanilla', label: 'Vanilla' }] },
{ path: 'gelato', type: 'textarea'}
]
});
The title
parameter lets you customize the title for each dialog type, improving the overall user experience. You can also define different path parameters to support multiple data paths within the editable area.
$uhuu.editDialog({
types: [
{ path: 'gelato', title: 'Gelato', type: 'select', options: [{ value: 'chocolate', label: 'Chocolate' },{ value: 'vanilla', label: 'Vanilla' }] },
{ path: 'comment', title: 'Comment', type: 'textarea'},
{ path: 'ingredients', title: 'Ingredients', type: 'markdown'},
]
});